design.ts
This file defines section design settings available to users in the Instant Site Editor. If some design settings like text size or font, or element color should be modifiable, you must add these settings to the design.ts
file.
Each setting is defined as the element_id
object with its properties inside. You can add multiple elements of the same type
to the file. If some of the content settings should also have design settings, use the same element_id
to link them.
How it looks in the Editor:
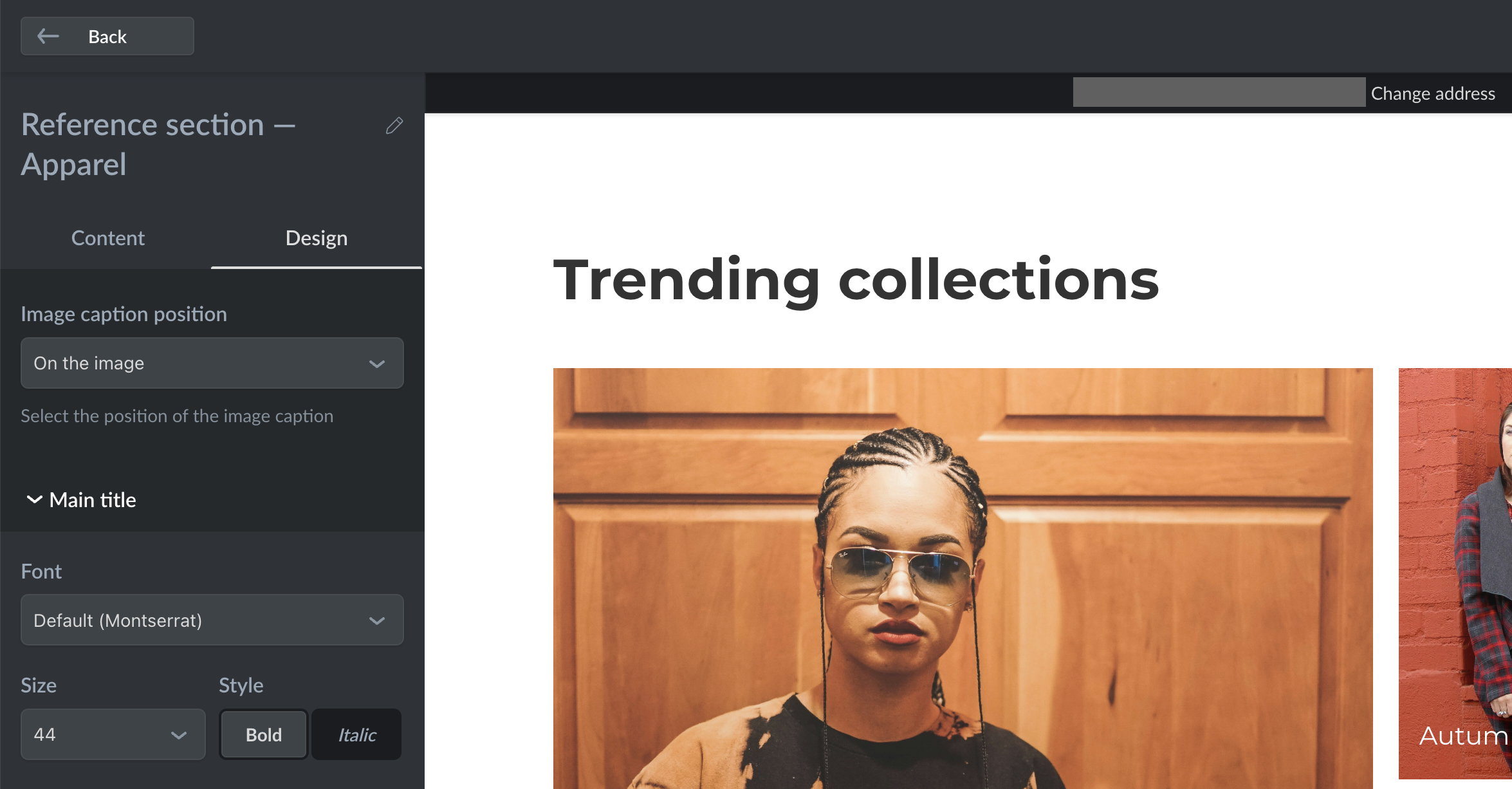
Find the full list of design types and their configs below:
TEXT
TEXT
Configuration for text elements.
Setting | Type | Description |
---|---|---|
type | string | Always TEXT . Works only with INPUTBOX and TEXTAREA element types. |
label | string | Element name. Use $label to add translations. |
colors | array of strings | An array of colors in the HEX code to choose from. Maximum: 7 colors. |
enableAlphaColor | boolean | Defines if the element can be transparent. |
enableAutoColor | boolean | Defines if Ecwid should select a matching color for the text automatically. |
hideVisibleToggle | boolean | Defines if the element visibility toggle is available in the Editor. |
sizes | array of numbers | An array of text sizes to choose from. Maximum: 7 sizes. |
hideSize | boolean | Defines if the element size menu is hidden in the Editor. Set true to hide. |
defaults | object | Default element settings applied when users install a section. |
The defaults
object contains the following fields:
Setting | Type | Description |
---|---|---|
font | string | Default text font. We recommend leaving global.fontFamily.body here to match the current store font. |
size | number | Default text size. |
bold | boolean | Defines if the text is bold by default. |
italic | boolean | Defines if the text is italicized by default. |
color | string | Default text color in HEX code. |
visible | boolean | Defines if the element is visible on the storefront. |
Code example for 'design.ts':
element_id: {
type: 'TEXT',
label: '$label.element_id.label',
colors: ['#FFFFFF66', '#0000004D', '#00000099', '#64C7FF66', '#F9947266', '#C794CD66', '#FFD17466'],
sizes: [12, 13, 14, 15, 16, 17, 18, 20],
defaults: {
font: 'global.fontFamily.body',
size: 16,
bold: false,
italic: true,
color: '#333',
}
BUTTON
BUTTON
Configuration for the button element.
Setting | Type | Description |
---|---|---|
type | string | Always BUTTON . Works only with the BUTTON element type. |
label | string | Element name. Use $label to add translations. |
colors | array of strings | An array of colors in the HEX code for the button background to choose from. Maximum: 7 colors. |
enableAlphaColor | boolean | Defines if the element can be transparent. |
enableAutoColor | boolean | Defines if Ecwid should select a matching color for the button automatically. |
hideVisibleToggle | boolean | Defines if the element visibility toggle is available in the Editor. |
hideSize | boolean | Defines if the element size menu is hidden in the Editor. Set true to hide. |
defaults | object | Default element settings applied when users install a section. |
The defaults
object contains the following fields:
Setting | Type | Description |
---|---|---|
appearance | string | Default button appearance. Available values: SOLID , OUTLINE , TEXT . |
shape | string | Default button shape. Available values: PILL , RECTANGLE , ROUND_CORNER . |
size | string | Default button size. Available values: SMALL , MEDIUM , LARGE . |
font | string | Default text font. We recommend leaving global.fontFamily.body here to match the current store font. |
color | string | Default text color in HEX code. |
visible | boolean | Defines if the element is visible on the storefront. |
Code example for 'design.ts':
element_id: {
type: 'BUTTON',
label: '$label.element_id.label',
colors: ['#FFFFFF66', '#0000004D', '#00000099', '#64C7FF66', '#F9947266', '#C794CD66', '#FFD17466'],
defaults: {
font: 'global.fontFamily.body',
size: 'MEDIUM',
appearance: 'OUTLINE',
shape: 'PILL',
color: '#333',
},
}
IMAGE
IMAGE
Configuration for the image uploader element.
Setting | Type | Description |
---|---|---|
type | string | Always IMAGE . Works only with the IMAGE element type. |
label | string | Element name. Use $label to add translations. |
colors | array of strings | An array of colors in the HEX code for the image background to choose from. Maximum: 7 colors. |
enableAlphaColor | boolean | Defines if the element can be transparent. |
hideVisibleToggle | boolean | Defines if the element visibility toggle is available in the Editor. |
defaults | object | Default element settings applied when users install a section. |
The defaults
object contains the following fields:
Setting | Type | Description |
---|---|---|
overlay | string | Default image overlay settings. Available settings: COLOR , GRADIENT , NONE . |
color | string/array | One (string type) or two (array with two strings inside) colors for default image overlay settings. |
Code example for 'design.ts':
element_id: {
type: 'IMAGE',
label: '$label.element_id.label',
colors: ['#FFFFFF66', '#0000004D', '#00000099', '#64C7FF66', '#F9947266', '#C794CD66', '#FFD17466'],
defaults: {
overlay: 'COLOR',
color: '#fff000',
},
}
TOGGLE
TOGGLE
Configuration for the toggle element.
Setting | Type | Description |
---|---|---|
type | string | Always TOGGLE . Works only with the TOGGLE element type. |
label | string | Element name. Use $label to add translations. |
description | string | Element description displayed in the Instant Site Editor under the element. |
defaults | object | Default element settings applied when users install a section. |
The defaults
object contains the following fields:
Setting | Type | Description |
---|---|---|
enabled | boolean | Defines if the toggle is enabled when users install the section. Set true to enable. |
Code example for 'design.ts':
element_id: {
type: 'TOGGLE',
label: '$label.element_id.label',
description: '$label.toggle.description',
defaults: {
enabled: true,
},
}
SELECTBOX
SELECTBOX
Configuration for the "selectbox" element that creates a drop-down list in the Editor.
Setting | Type | Description |
---|---|---|
type | string | Always SELECTBOX . Works only with the SELECTBOX element type. |
label | string | Element name. Use $label to add translations. |
description | string | Description under the drop-down. |
options | array of objects | List of options available in the drop-down list. |
defaults | object | Default element settings applied when users install a section. |
The options
is an array where each object contains the following fields:
Setting | Type | Description |
---|---|---|
value | string | Option value. |
label | string | Text label for the option value. |
The defaults
object contains the following fields:
Setting | Type | Description |
---|---|---|
value | string | Default option value selected when users install a section. If not specified, users see placeholder text instead. |
Code example for 'design.ts':
element_id: {
type: 'SELECTBOX',
label: '$label.element_id.label',
description: '$label.element_id.description',
options: [
{
value: 'one',
label: '$label.element_id.one.label',
},
{
value: 'two',
label: '$label.element_id.two.label',
},
],
defaults: {
value: 'two',
},
}
BACKGROUND
BACKGROUND
Configuration for the section background which is not an element type defined in the 'content.ts' file.
Setting | Type | Description |
---|---|---|
type | string | Always BACKGROUND . |
label | string | Element name. Use $label to add translations. |
colors | array of strings | An array of colors in the HEX code for the background to choose from. Maximum: 7 colors. |
enableAlphaColor | boolean | Defines if the element can be transparent. |
enableAutoColor | boolean | Defines if Ecwid should select a matching color for the background automatically. |
defaults | object | Default element settings applied when users install a section. |
The defaults
object contains the following fields:
Setting | Type | Description |
---|---|---|
style | object | Background type. Available values: COLOR (single color background) or GRADIENT (background with gradient). |
color | string/array | One (string type) or two (array with two strings inside) colors for default background color settings. |
Code example for 'design.ts':
element_id: {
type: 'BACKGROUND',
label: '$label.element_id.label',
colors: ['#FFFFFF66', '#0000004D', '#00000099', '#64C7FF66', '#F9947266', '#C794CD66', '#FFD17466'],
defaults: {
style: 'COLOR',
color: '#fff000',
},
}
Updated 3 months ago